- Documentation
- Video Conference Kit
- Quick start
Quick start
Integrate the SDK
Add ZegoUIKitPrebuiltVideoConference as dependencies
Run the following code in your project root directory:
flutter pub add zego_uikit_prebuilt_video_conference
Import the SDK
Now in your Dart code, import the prebuilt Video Conference Kit SDK.
import 'package:zego_uikit_prebuilt_video_conference/zego_uikit_prebuilt_video_conference';
Using the ZegoUIKitPrebuiltVideoConference in your project
- Go to ZEGOCLOUD Admin Console, get the
appID
andappSign
of your project. - Specify the
userID
anduserName
for connecting the Video Conference Kit service. - Create a
conferenceID
that represents the video conference you want to start.
userID
andconferenceID
can only contain numbers, letters, and underlines (_).- Using the same
conferenceID
will enter the same video conference.
class VideoConferencePage extends StatelessWidget {
final String conferenceID;
const VideoConferencePage({
Key? key,
required this.conferenceID,
}) : super(key: key);
@override
Widget build(BuildContext context) {
return SafeArea(
child: ZegoUIKitPrebuiltVideoConference(
appID: YourAppID, // Fill in the appID that you get from ZEGOCLOUD Admin Console.
appSign: YourAppSign, // Fill in the appSign that you get from ZEGOCLOUD Admin Console.
userID: 'user_id',
userName: 'user_name',
conferenceID: conferenceID,
config: ZegoUIKitPrebuiltVideoConferenceConfig(),
),
);
}
}
Now, you can start a video conference by navigating to this VideoConferencePage
.
Configure your project
- Android:
1. modify the
If your project is created with Flutter 2.x.x, you will need to open thecompileSdkVersion
your_project/android/app/build.gradle
file, and modify thecompileSdkVersion
to 33.
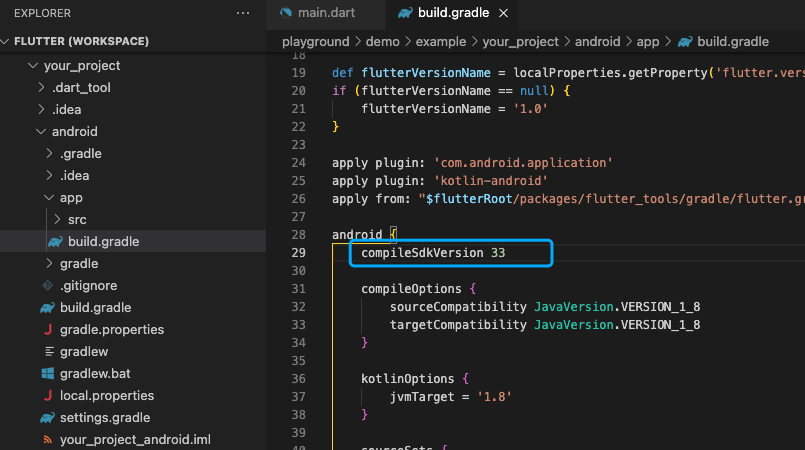)
2. Add app permissions.
Open the file your_project/app/src/main/AndroidManifest.xml
, and add the following code:
<uses-permission android:name="android.permission.ACCESS_WIFI_STATE" />
<uses-permission android:name="android.permission.RECORD_AUDIO" />
<uses-permission android:name="android.permission.INTERNET" />
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" />
<uses-permission android:name="android.permission.CAMERA" />
<uses-permission android:name="android.permission.BLUETOOTH" />
<uses-permission android:name="android.permission.MODIFY_AUDIO_SETTINGS" />
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
<uses-permission android:name="android.permission.READ_PHONE_STATE" />
<uses-permission android:name="android.permission.WAKE_LOCK" />
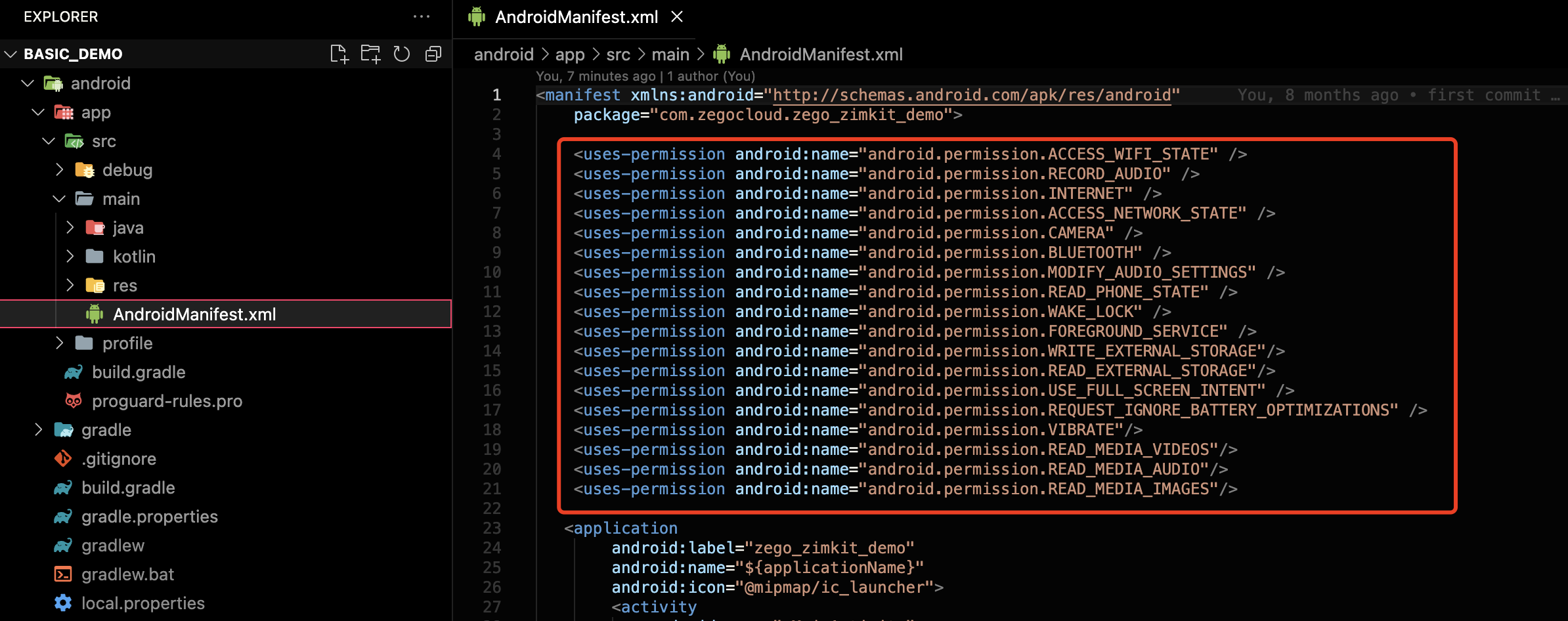)
3. Prevent code obfuscation.
To prevent obfuscation of the SDK public class names, do the following:
a. In your project's your_project > android > app
folder, create a proguard-rules.pro
file with the following content as shown below:
-keep class **.zego.** { *; }
b. Add the following config code to the release
part of the your_project/android/app/build.gradle
file.
proguardFiles getDefaultProguardFile('proguard-android.txt'), 'proguard-rules.pro'
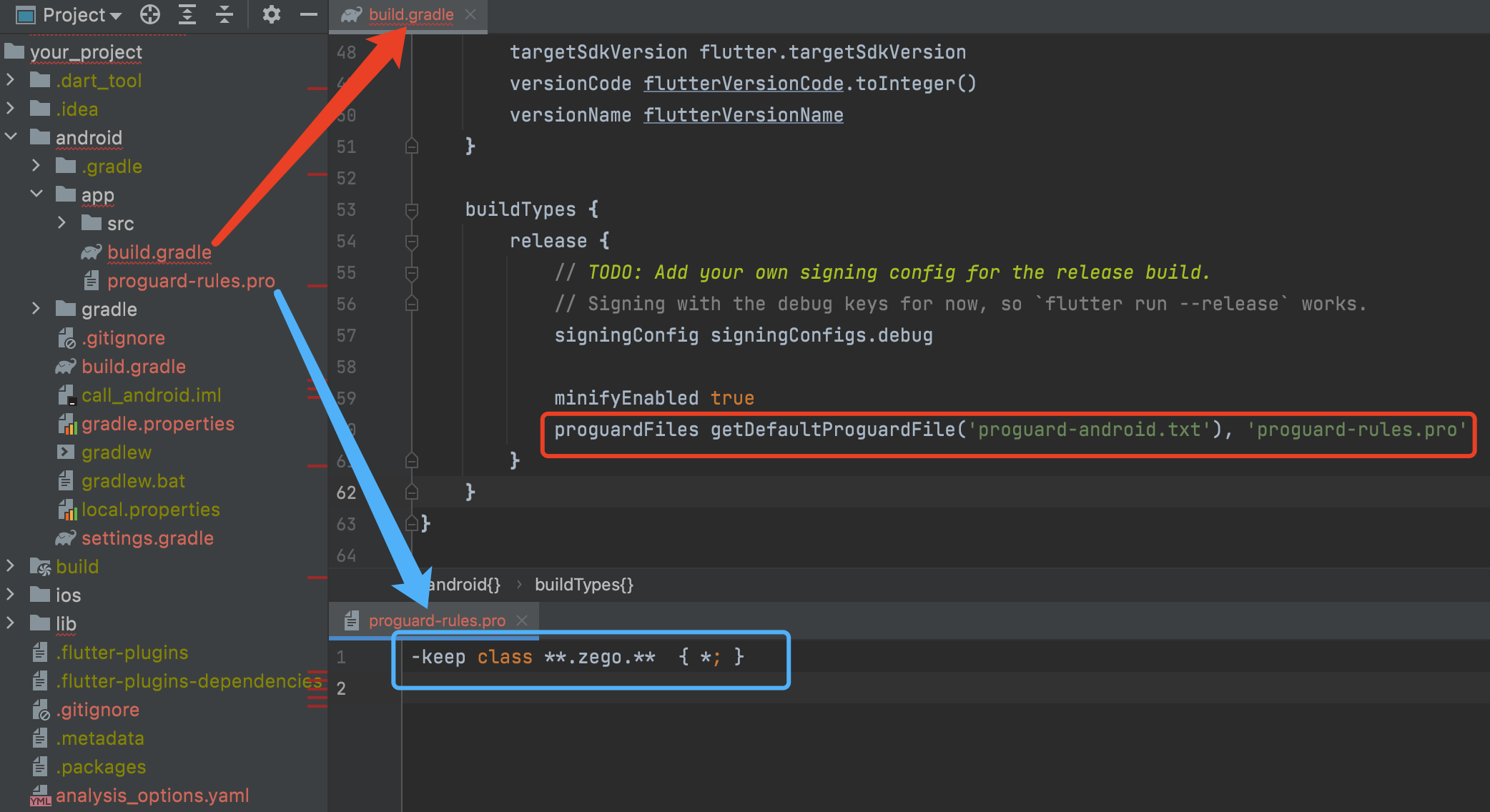)
- iOS:
1. Add app permissions.
a. Open the your_project/ios/Podfile
file, and add the following to the post_install do |installer|
part:
# Start of the permission_handler configuration
target.build_configurations.each do |config|
config.build_settings['GCC_PREPROCESSOR_DEFINITIONS'] ||= [
'$(inherited)',
'PERMISSION_CAMERA=1',
'PERMISSION_MICROPHONE=1',
]
end
# End of the permission_handler configuration
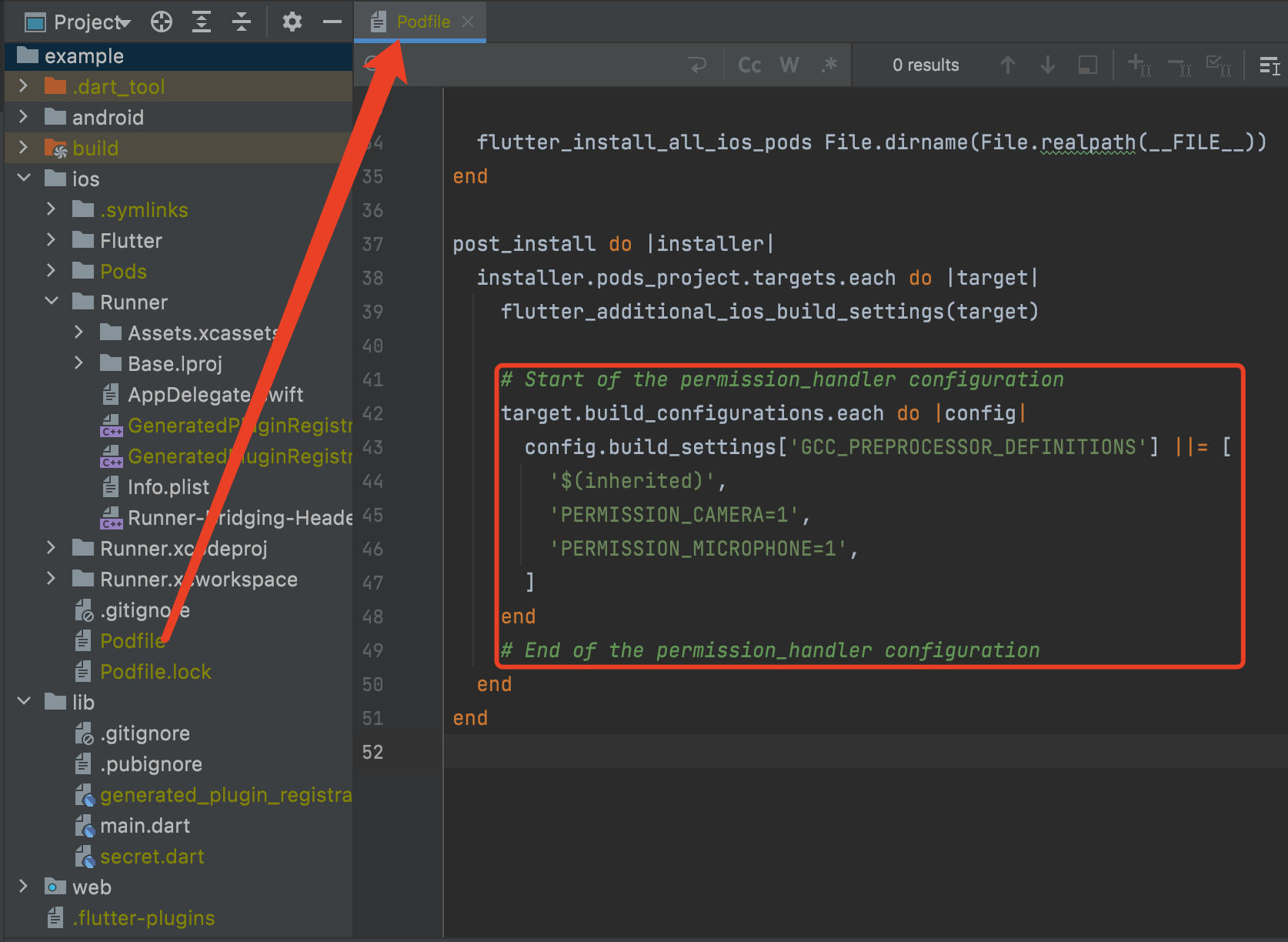)
b. Open the your_project/ios/Runner/Info.plist
file, and add the following to the dict
part:
<key>NSCameraUsageDescription</key>
<string>We require camera access to connect</string>
<key>NSMicrophoneUsageDescription</key>
<string>We require microphone access to connect</string>
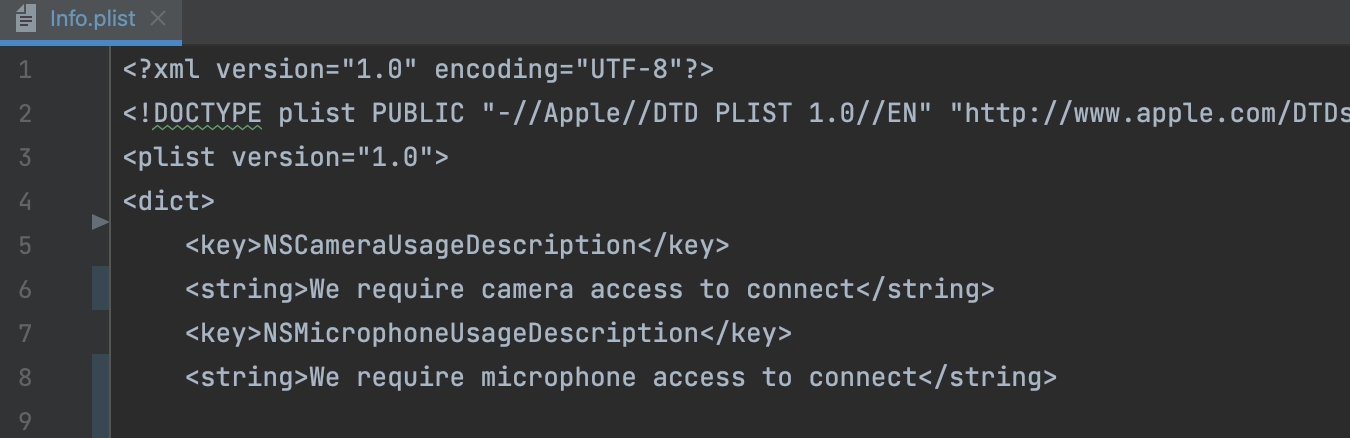)
Run & Test
Now you have finished all the steps!
You can simply click the Run or Debug to run and test your App on your device.
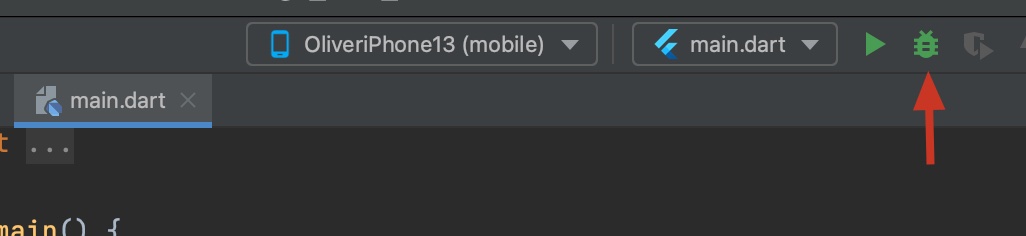)