- Documentation
- Collaborative Whiteboard
- Server APIs
- API Call Method
API Call Method
1 Request Structure
1.1 Service Endpoint
The ZEGO server API supports global access nearby, using the global unified domain name ${PRODUCT}-api.zego.im to resolve it by region.
At the same time, regional domain names are provided. The list of supported domain names is as follows:
Geographical Area | Access Domain Name |
---|---|
Mainland China (Shanghai) |
${PRODUCT}-api-sha.zego.im |
Hong Kong, Macau and Taiwan (Hong Kong) |
${PRODUCT}-api-hkg.zego.im |
Europe (Frankfurt) |
${PRODUCT}-api-fra.zego.im |
United States (California) |
${PRODUCT}-api-lax.zego.im |
Asia Pacific (Mumbai) |
${PRODUCT}-api-bom.zego.im |
The ${PRODUCT} in the domain name is each product provided by ZEGO, and the corresponding domain names are as follows:
Product | ${PRODUCT} Value | Access Domain Name |
---|---|---|
Cloud Communication | rtc | rtc-api.zego.im |
Interactive whiteboard | whiteboard | whiteboard-api.zego.im |
1.2 Communication Protocol
All interfaces of the ZEGO server API communicate via HTTPS to provide secure communication services.
1.3 Request Method
The ZEGO server API supports the following HTTP request methods:
- GET
- POST
All request parameters (including public parameters and business parameters) are placed in Query, using the GET request method. The special and complex API parameters are placed in the Body, and the POST request method is used.
2 Public Parameters
This section introduces the public parameters used by developers when calling the ZEGO server API, including public request parameters and public return parameters.
2.1 Public Request Parameters
Common request parameters are request parameters that every interface needs to use.
Parameters | Type | Required | Description |
---|---|---|---|
AppId |
Uint32 |
Yes |
AppId, the user's unique credential assigned by ZEGO. |
Signature |
String |
Yes |
Signature, please refer to 3 signature mechanism for signature generation.
|
SignatureNonce | String |
Yes |
Random string. |
SignatureVersion |
String |
Yes |
Signature version number, the default value is 2.0. |
Timestamp |
Int64 |
Yes |
Unix timestamp, in seconds. An error of up to 10 minutes is allowed.
|
IsTest | String |
No |
Whether it is a test environment.
|
Example request:
https://rtc-api.zego.im/?Action=ForbidLiveStream
&AppId=1234567890
&SignatureNonce=15215528852396
&Timestamp=1234567890
&Signature=Pc5WB8gokVn0xfeu%2FZV%2BiNM1dgI%3D
&SignatureVersion=2.0
&IsTest=false
2.2 Public Return Parameters
The API return results are in a uniform format, and the returned data format is JSON.
Every time the interface is called, no matter whether it is successful or not, the public parameters will be returned.
Parameters | Type | Description |
---|---|---|
Code |
Number |
Return code. |
Message |
String |
Description of the operation result. |
RequestId |
String |
Request ID. |
Data |
- |
Response data. |
Return example:
{
"Code":0,
"Data":{
"MessageId":"1_1611647493487_29"
},
"Message":"success",
"RequestId":"2237080460466033406"
}
3 Signature Mechanism
To ensure the security of API calls, the ZEGO server will authenticate each API access request. When developers call the API, they need to include signature information in the request.
A new signature needs to be generated every time the interface is called.
3.1 Key Acquisition
Signature uses AppID and ServerSecret for symmetric encryption to verify the identity of the sender of the request. AppID is used to identify the identity of the visitor, and ServerSecret is used to encrypt the signature string and the key used by the server to verify the signature string. It must be strictly confidential to prevent leakage.
Developers can go to ZEGO console to obtain the AppID and ServerSecret, and view related information in "My Project > Project > Configuration > Basic Information".
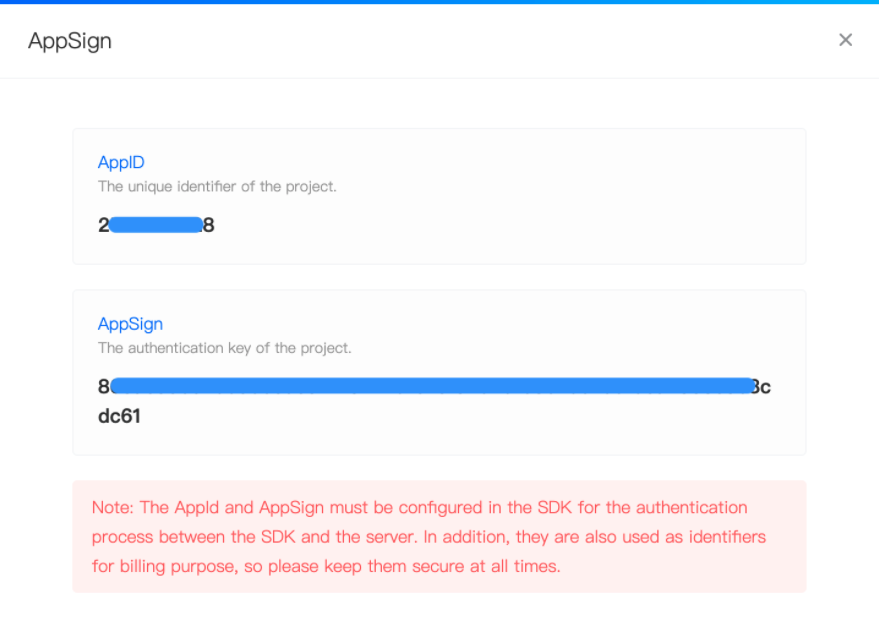)
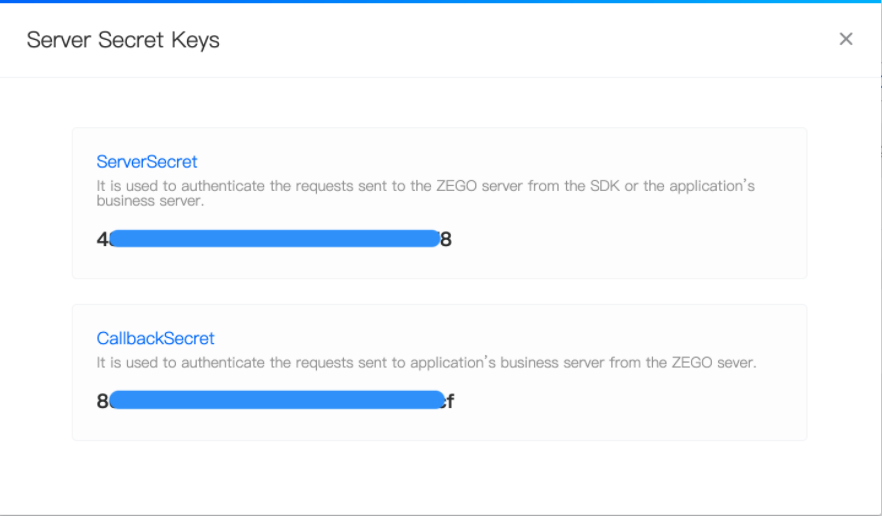)
3.2 Signature Generation
- Signature parameter description
Parameters | Meaning |
---|---|
AppId |
App ID. The AppId in the public parameters is obtained from ZEGO console. |
SignatureNonce |
Random string. For SignatureNonce in the public parameters, the generation algorithm can refer to the following signature example. |
ServerSecret |
Application secret key, obtained from ZEGO console. |
Timestamp |
Current Unix timestamp, in seconds. The generation algorithm can refer to the signature example below, and the error of up to 10 minutes is allowed. |
- Signature Generation Algorithm
Signature = md5(AppId + SignatureNonce + ServerSecret + Timestamp)
- Signature String Format
The signature uses hex encoding (small letters), with a length of 32 characters.
3.3 Signature Example
ZEGO provides signature sample codes in a variety of programming languages, and developers can refer to them according to the actual situation.
Go signature example
import (
"crypto/md5"
"crypto/rand"
"encoding/hex"
"fmt"
"log"
"time"
)
// Signature=md5(AppId + SignatureNonce + ServerSecret + Timestamp)
func GenerateSignature(appId uint32, signatureNonce string, serverSecret string, timestamp int64) (Signature string){
data := fmt.Sprintf("%d%s%s%d", appId, signatureNonce, serverSecret, timestamp)
h := md5.New()
h.Write([]byte(data))
return hex.EncodeToString(h.Sum(nil))
}
func main() {
/*Generate hexadecimal random string (16 bits)*/
nonceByte := make([]byte, 8)
rand.Read(nonceByte)
signatureNonce := hex.EncodeToString(nonceByte)
log.Printf(signatureNonce)
appId := 12345 //Use your appId and serverSecret
serverSecret := "9193cc662a4c0ec135ec71fb57194b38"
timestamp := time.Now().Unix()
/* appId:12345
signatureNonce:4fd24687296dd9f3
serverSecret:9193cc662a4c0ec135ec71fb57194b38
timestamp:1615186943 2021/03/08 15:02:23
signature:43e5cfcca828314675f91b001390566a
*/
log.Printf("signature:%v", GenerateSignature(uint32(appId), signatureNonce, serverSecret, timestamp))
}
Python signature example
# -*- coding: UTF-8 -*-
import secrets
import string
import hashlib
import time
#Signature=md5(AppId + SignatureNonce + ServerSecret + Timestamp)
def GenerateSignature(appId, signatureNonce, serverSecret, timestamp):
str1 = str(appId) + signatureNonce + serverSecret + str(timestamp)
hash = hashlib.md5()
hash.update(str1.encode("utf8"))
signature = hash.hexdigest()
return signature
def main():
#Generate hexadecimal random string (16 bits)
signatureNonce = secrets.token_hex(8)
#Use your appId and serverSecret
appId = 12345
serverSecret = "9193cc662a4c0ec135ec71fb57194b38"
#Get 10-digit unix timestamp
timestamp = int(time.time())
print(GenerateSignature(appId,signatureNonce,serverSecret,timestamp))
if __name__ =='__main__':
main()
Java signature example
import java.security.NoSuchAlgorithmException;
import java.security.SecureRandom;
public class md5{
/**
* Byte array to hexadecimal
* @param bytes byte array to be converted
* @return Hex string after conversion
*/
public static String bytesToHex(byte[] bytes) {
StringBuffer md5str = new StringBuffer();
//Change each byte of the array to hexadecimal and connect to md5 string
int digital;
for (int i = 0; i <bytes.length; i++) {
digital = bytes[i];
if (digital <0) {
digital += 256;
}
if (digital <16) {
md5str.append("0");
}
md5str.append(Integer.toHexString(digital));
}
return md5str.toString();
}
// Signature=md5(AppId + SignatureNonce + ServerSecret + Timestamp)
public static String GenerateSignature(long appId, String signatureNonce, String serverSecret, long timestamp){
String str = String.valueOf(appId) + signatureNonce + serverSecret + String.valueOf(timestamp);
String signature = "";
try{
//Create an object that provides information digest algorithm, initialized as md5 algorithm object
MessageDigest md = MessageDigest.getInstance("MD5");
//Get the byte array after calculation
byte[] bytes = md.digest(str.getBytes("utf-8"));
//Change each byte of the array to hexadecimal and connect to md5 string
signature = bytesToHex(bytes);
}catch (Exception e) {
e.printStackTrace();
}
return signature;
}
public static void main(String[] args){
//Generate hexadecimal random string (16 bits)
byte[] bytes = new byte[8];
SecureRandom sr = null;
//Use SecureRandom to obtain a high-strength secure random number generator
try{
sr = SecureRandom.getInstanceStrong();
}catch(Exception e){
e.printStackTrace();
}
sr.nextBytes(bytes);
String signatureNonce = bytesToHex(bytes);
long appId = 12345L; //Use your appId and serverSecret, add uppercase L or lowercase l after the number to indicate the long type
String serverSecret = "9193cc662a4c0ec135ec71fb57194b38";
long timestamp = System.currentTimeMillis() / 1000L;
System.out.println(GenerateSignature(appId,signatureNonce,serverSecret,timestamp));
}
}
PHP signature example
<?php
function GenerateSignature($appId, $signatureNonce, $serverSecret, $timestamp)
{
$str = $appId.$signatureNonce.$serverSecret.$timestamp;
$signature = md5($str);
return $signature;
}
//Generate hexadecimal random string (16 bits)
$signatureNonce = bin2hex(random_bytes(8));
//Use your appId and serverSecret
$appId = 12345;
$serverSecret = "9193cc662a4c0ec135ec71fb57194b38";
$timestamp = time();
$signature = GenerateSignature($appId, $signatureNonce, $serverSecret, $timestamp);
echo $signature;
?>
Node.js signature example
const crypto = require('crypto');
//Signature=md5(AppId + SignatureNonce + ServerSecret + Timestamp)
function GenerateUASignature(appId, signatureNonce, serverSecret, timeStamp){
const hash = crypto.createHash('md5'); //Specify the use of the MD5 algorithm in the hash algorithm
var str = appId + signatureNonce + serverSecret + timeStamp;
hash.update(str);
//hash.digest('hex') indicates that the output format is hexadecimal
return hash.digest('hex');
}
var signatureNonce = crypto.randomBytes(8).toString('hex');
//Use your appId and serverSecret
var appId = 12345;
var serverSecret = "9193cc662a4c0ec135ec71fb57194b38";
var timeStamp = Date.now();
console.log(GenerateUASignature(appId, signatureNonce, serverSecret, timeStamp));
3.4 Signature Failure
There are the following return codes for signature failures, please deal with them according to the actual situation.
Return code | Description |
---|---|
100000004 | The signature has expired. |
100000005 | Signature error. |