- Documentation
- Live Streaming
- Develop your app
- Live Streaming
- Enhance basic livestream
- Set up common livestream config
Set up common video config
Introduction
ZEGOCLOUD supports setting up the video configuration during a real-time video call or live streaming, such as video capture and video encoding output resolution, video frame rate, bitrate, view mode, and mirror mode.
Setting the proper video resolution, frame rate, and bitrate to provide a better experience in audio and video scenarios. Setting the appropriate view modes to provide a personalized video display mode.
Basic concepts
- Resolution:
- Video resolution: The number of pixels contained in each frame. The video resolution is often expressed in PPI.
- Capture resolution: The total amount of pixels captured by a camera.
- Encoder resolution: The resolution of the encoded image.
- Bitrate (bps): The number of bits that are conveyed or processed per unit of time. The bitrate is expressed in the unit bit per second unit.
- Frame rate (fps): The frequency (rate) at which consecutive images (frames) are captured or displayed. The frame rate is often expressed in frames per second or fps.
Prerequisites
Before you begin, make sure you complete the following:
Create a project in ZEGOCLOUD Admin Console and get the AppID and AppSign of your project.
Refer to the Quick Start doc to complete the SDK integration and basic function implementation.
Set up the video configuration
Use default values
If you don't want to manually set the video configuration, you can use the sets of default values provided by the ZEGO Express SDK, the default values of ZegoVideoConfigPreset are as follows:
ZegoVideoConfigPreset | Capture resolution (Width × Height) |
Encoder resolution (Width × Height) |
Frame rate (fps) |
Bitrate (kbps) |
---|---|---|---|---|
Preset180P |
180 × 320 |
180 × 320 |
15 |
300 |
Preset270P |
270 × 480 |
270 × 480 |
15 |
400 |
Preset360P |
360 × 640 |
360 × 640 |
15 |
600 |
Preset540P |
540 × 960 |
540 × 960 |
15 |
1200 |
Preset720P |
720 × 1280 |
720 × 1280 |
15 |
1500 |
Preset1080P |
1080 × 1920 |
1080 × 1920 |
15 |
3000 |
The sample code for using default values is shown below:
ZegoVideoConfig videoConfig = ZegoVideoConfig.preset(ZegoVideoConfigPreset.Preset1080P);
ZegoExpressEngine.instance.setVideoConfig(videoConfig);
Customize the video configuration
You will need to set up the video configuration before the stream publishing starts (startPublishingStream
) or local video preview starts (startPreview
).
Only the encoder resolution and bitrate can be modified after stream publishing.
To set up the video configuration, call the setVideoConfig
method. If you don't customize your video configuration, ZEGO uses the default values as shown below:
Item | Default value |
---|---|
Resolution | 360p |
Bitrate | 600 kbps |
Framerate | 15 fps |
The following sample code is used for setting the video capture resolution to 360p, encoder resolution to 360p, bitrate to 600 kbps, and frame rate to 15 fps:
ZegoVideoConfig videoConfig = ZegoVideoConfig(360, 640, 360, 640, 15, 600, ZegoVideoCodecID.Default);
// Setting up video Configuration.
ZegoExpressEngine.instance.setVideoConfig(videoConfig);
The width and height resolutions of the mobile terminal are opposite to those of the PC terminal. For example, the 360p resolution of the mobile terminal is 360x640, while the 360P resolution of the PC terminal is 640x360.
Set the video view mode
To set the video view mode, you will need to stop the local video preview or stop stream playing first; Otherwise, the settings won't take effect.
To set the video view mode, modify the viewMode
property of the ZegoCanvas
obeject.
Currently, the following three video rendering and filling modes are supported:
Enumerated value | Description |
---|---|
AspectFit | Uniform scaling, the image may have black edges. |
AspectFill | Uniform scaling and filling the entire View, and images may be cropped. |
ScaleToFill | Fill the entire View, and the image may be stretched. |
The effects of the three video rendering and filling modes are as shown below:
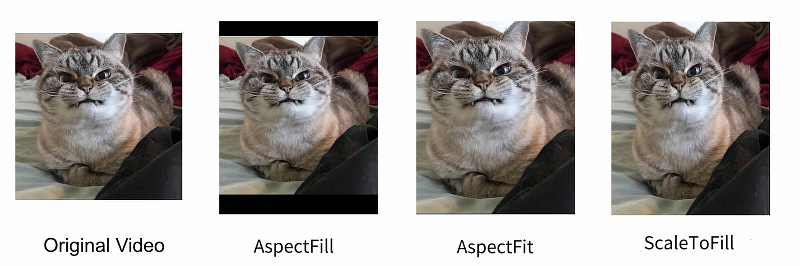)
The resolution of the image shown above is 320 x 390 (width x height), and the video resolution is 340 x 340 (width x height).
As shown above, the height of the original video is equal to the width, while the height of the image is greater than the width. Therefore, in the
ASPECT_FIT
view mode, black edges appear on the top and bottom of the video. If the width of the image is larger than the height, black edges will appear on the left and right sides of the video inASPECT_FIT
view mode.
The following sample code is used for setting the view mode to ASPECT_FIT
and starting the local video preview:
// previewID for the ID obtained by createPlatformView or createTextureRenderer
// Set the display mode to AspectFit
previewCanvas = new ZegoCanvas(previewID, viewMode: ZegoViewMode.AspectFit);
// Start the local video preview.
ZegoExpressEngine.instance.startPreview(previewCanvas);
Set the mirror mode
This mode can also be set during stream publishing and take effect immediately.
To mirror the video you want to publish or mirror the video in a local video preview, call the setVideoMirrorMode
method before or after the stream publishing.
Currently, the following four mirror modes are supported:
Enumeration value | Description |
---|---|
NoMirror | Neither Capturer (local video preview) nor stream player gets mirrored images. |
OnlyPreviewMirror | The image is mirrored only in the local video preview. This mode is used by default. |
OnlyPublishMirror | Only stream player is expected to see mirrored images. |
BothMirror | Capturer (local video preview) and stream player are expected to see mirrored images. |
The effects of the four mirror modes are as shown below:
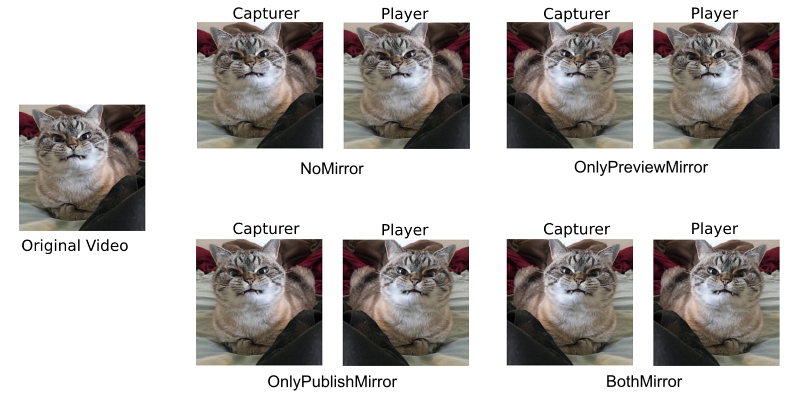)
The following sample code is used for setting the mirror mode for the stream player without mirroring the local video preview:
ZegoExpressEngine.instance.setVideoMirrorMode(ZegoVideoMirrorMode.OnlyPublishMirror);